I once worked on a medical project which required intense Unit Testing in C#. A problem I encountered is that all of the critical sections of the code can't be accessed or required a massive amount of object dependencies. The dilemma I was in drove me to search for a new soluion. Good thing I found TypeMock Isolator.
TypeMock Isolator is a mocking framework that can take control of function calls regardless if it is accessible or not such as privately declared functions and etc. This Framework is center-focused on white box testing legacy code; although, can be used for black box testing.
"context" can be use to :
1.) Get method’s instance
2.) Get/Set arguments
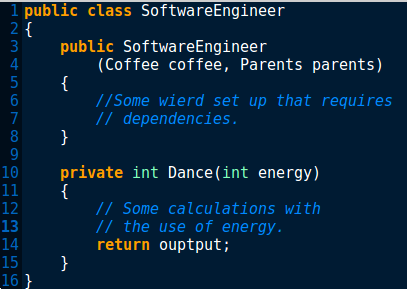

TypeMock Isolator is a mocking framework that can take control of function calls regardless if it is accessible or not such as privately declared functions and etc. This Framework is center-focused on white box testing legacy code; although, can be used for black box testing.
Why use it?
- Code not Written using SOLID Principles
- Tested code is not following TDD(Test Driven Development)
- Testing legacy code
- Unrefactorable tested code
- Excellent for mission critical code.
Mock Instantiation
Using Typemock Isolator, object instantiations can be ignored.
- Ignore Constructor
- Ignore Base Class
Mock Behaviour
A powerful feature of this framework is its ability to switch out function calls regardless of its access modifier.
- Non-Public Method
"context" can be use to :
1.) Get method’s instance
2.) Get/Set arguments
- Public Method
- Properties
Invoke!
Another useful feature is being able to Invoke inaccessible functions so it can be tested.
- Invoke NonPublic methods
- Fire events
Example 1
This example shows that private functions can be invoked and tested without having to care about dependencies in constructor.
Code to Test
Unit Test
Example 2
This example shows that you don't have to think about dependencies on the function calls inside your unit on test. In this case, Coolness function's behaviour is being swaped by a dummy lambda function.
Code to Test
Unit Test
Example 3
Lastly, let us look into this really interesting test problem. Some critical systems will definitely need to have some variables set to a certain value to operate correctly. In the constructor, in the middle of operation, _hunger needs to be in a certain value before and after SearchFood() function. Normally, this is almost impossible to do. We can test SearchFood() function as a hook to extract the current state of _hunger.
Code to Test
Unit Test
Conclusion
If you are stuck in a similar dilemma as I did wherein I could not access anything, Here is the solution to the problem! This framework can save you lots and lots of time.
Here is the official website of Typmock.
Comments
Post a Comment